11 Reasons to use Spring Framework
Becoming a Java programmer is a great choice.
Over 20 years of development, Java has left many imprints, good and bad. If you consider Java as an important platform to build Enterprise Software, Spring makes up a small part of that platform.
When Spring was born, its mission was to replace enterprise-class Java technologies, which were quite cumbersome and heavy as EJB. Spring proposes a softer, more compact solution than EJB by pumping POJO power that is only available to EJB and its brothers. Gradually EJB and J2EE also improved in Spring's direction: EJB also used POJO, implemented DI (Dependency Injection) and AOP (Aspect-Oriented Programming) ideas.
Although J2EE (or JEE) can catch up with Spring, Spring is constantly developing and reaching out to the new J2EE lands that are just toddling or never set foot in: mobile development, social API integration, NoSQL database, cloud computing, big data … Spring's future is really bright.
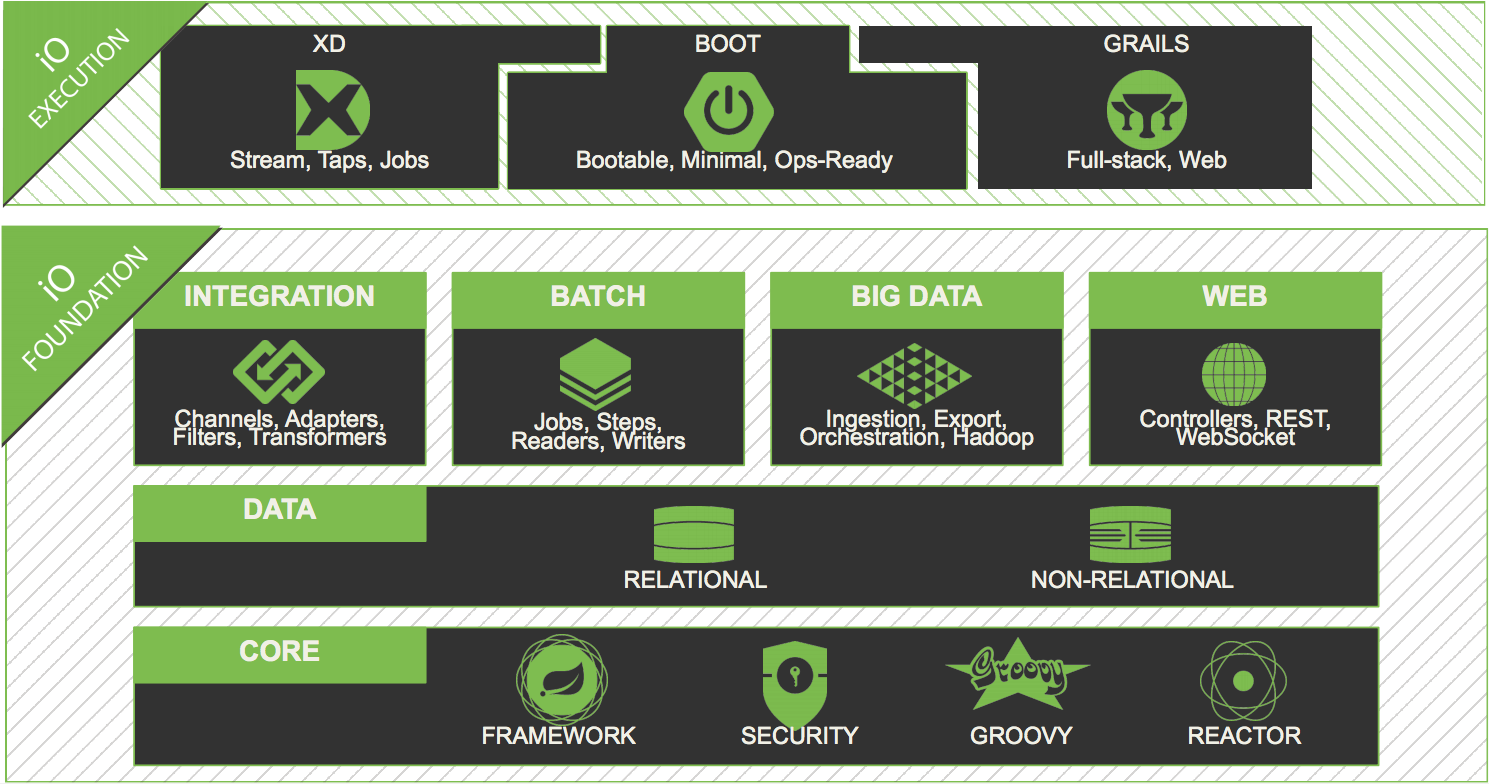
I have been a Spring developer since 2004. Since then, I have been using Spring for every project I participate in. Spring includes a lot of modules and each project I apply is one or more suitable modules.
In this article, I listed 11 reasons to use Spring for your project. I will try to help you get an overview of Spring modules as well as how to apply them. All are drawn from my real combat experience.
You may be unfamiliar with some technologies but it is important that you understand the problem that technology is addressing.
1. Build Web Application with Spring:
With many Java developers, web application development is their main goal. If you have been aiming for this goal, you will clearly see 3 challenges to overcome: state management, workflow, validation. One more unhappy thing, HTTP is a stateless design, so passing these three challenges is never simple.
Spring MVC is a framework built on Spring platform. It still preserves the essence of Spring and is an effective tool to assist you in this regard. Based on the classic MVC model, Spring MVC will help you build powerful and flexible web applications.
Although I did not start my Spring developer career with Spring MVC, most of the projects I participated in later needed it.
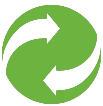
If you need a webful stateful type, try Spring Web Flow. Spring Web Flow helps you build a wizard – a set of windows that users must browse sequentially before obtaining data. Spring Web Flow helps you avoid storing too much data temporarily.
With Spring Web Flow, you can try a project to book flights, train tickets.
2. Access to RESTful Service:
For this part, get familiar with Spring HATEOAS. HATEOAS is a REST application architecture constraint. See more about HATEOAS here .
HATEOAS = Hypermedia As The Engine Of Application State.

I will try a brief explanation about HATEOAS. I understand its concept but I have not applied HATEOAS to my project ever.
A web service developer will provide a "contract" to the consumer of that service before the consumer calls to the service.
In the world of SOAP, this is almost the same as creating an interface, thanks to that interface the client knows which method can be used.
In the REST world, we build URLs with relevant data, we still need to know what information the server is "expecting".
In Hypermedia's world, the resource identifier component will provide elements related to the information it needs. These factors can be regarded as conventions for interaction between the two parties in the future.
Jersey is also an open source web service built on the Java platform. The construction of Jersey's web service follows the JSR specification.
Converting APIs from Spring to Jersey and vice versa is relatively easy.
Jersey only focuses on REST, Jersey cannot support a JSP webpage while Spring MVC brings you both excellently. If the client wants to use jQuery in JSP webpage, Spring MVC fully satisfies. Even if you want to use any MVC JavaScript framework, Spring MVC still supports you. In my opinion, when you are confused between Spring MVC and other solutions, follow Spring MVC.
Spring MVC provides a serenity as well as a coherent structure for the servlet programming model. It separates the model from the view (Actually, this problem was solved by Struts MVC a long time ago. Spring MVC just followed a bit)
Javascript frameworks MVC have some similarities to Spring MVC but they are applied to Javascript layer. With Javascript MVC frameworks, you can build web pages independent of server logic.
Two tiers built from Java and Javascript will communicate and exchange data with the server via RESTful URLs (RESTful URLs).
3. Spring Security and security issues:
With all projects involved in web development, I need Spring Security. Here are 2 minutes to introduce Spring Security:
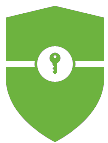
Spring Security will interceipt URLs according to a certain pattern (for example, URLs with the form "/ user" will be accessible to all users, and the "admin" format will only be available to some individuals) there.
Thanks to Spring Security, you can paste an annotation on methods to serve security. I will spend an article on this issue.
Spring Security provides both authentication and authorization mechanisms:
Authentication – authentication : determine who you are
Decentralization – authorization: limit your actions
When starting a new project, I integrate Spring Security and use in-memory authentication .
With this mechanism, you will make available a bunch of usernames, passwords and permissions, insert them into an xml file or java … Spring Security will allow you to access with a corresponding username and password, after access, You will be assigned the right permissions. Later, you can change the authentication and authorization mechanisms without affecting the business logic of the system.
4. Manipulate in database with Spring
To work with databases, I often use Spring ORM and hibernate.
ORM = Object Relational Mapping – A mechanism to represent data tables in the form of Java object and vice versa. Hibernate and myBatis are the two most popular ORM frameworks. An interesting fact, before the iPhone and iPad became popular, myBatis was known as iBatis .
If you have to handle a large amount of bloated JDBC code, go to Spring JDBC. Spring JDBC will help significantly reduce traditional JDBC code blocks through Templates
I have encountered cases of using hibernate for ORM but have to call a SQL procedure from the Oracle database administration system. Because hibernate only accepts "appropriate" procedure types, I will use Spring JDBC to call this procedure if hibernate does not accept it.
If you are working on a new project, try Spring Data …. With Spring Data, you don't need to write CRUD operations. They are built-in, I will explain this in another article. I have incorporated Spring Data with Oracle and MongoDB, everything works very smoothly. Spring Data also supports NoSQL databases and graphs databases such as Redis, Neo4j, Cassandra, Couchbase ….
CRUD operation = CR eate, U pdate, D elete. See more about CRUD here
5. Spring Batch
Spring Batch provides a set of reusable functions (reusable functions). These functions play a crucial role when processing a large number of records in the database.
I have encountered cases where database queries take up to 1 hour to complete (I will explain why). And of course you can't force users to wait that time to get the results they want.
In a project I participated in related auditing, every operation on the database affected millions of records, in this case, we used Spring Batch.
At that time, each request will trigger a Spring Batch Job and immediately a Job ID will be returned. I can use that Job ID to control the status of the processing process and notify the user of the progress of the job. Notifications for users may appear as an email notification or you can use web sockets to shoot notifications directly to users when they are online.
Spring Batch also provides you with a solution to automatically generate notifications when the status of the system changes. …
Spring Batch will ensure data integrity and consistency.
6. Spring Integration and peripheral systems:
In my recent project, the partner sends reports through the web service in XML format. On average, we receive 100,000 XML files in 6 months. These XML files are not too big, but the problem here is that it is impossible to determine when the files will be sent. Therefore, the goal is to have an automatic "catch" of these files as soon as they are sent, then proceed with the pre-processing and then transfer them to the system.

It is a common scenario when you build a service depending on the external system.
In this case, we chose Spring Integration. We install a poller file – take that place to store the files sent by the client. When any "fly" file arrives, an event is automatically triggered, processing operations are performed and transferred to the system.
Of course the code that handles these files is completely independent of the rest of the system. As a result, our system does not depend entirely on the partner system. Besides, testing the modules to receive this file is also much easier.
7. Spring Test
Spring does not stand outside the TDD trend. It provides a module for testing applications – Spring Test
In this module, with Unit Test, you will have a set of mock object implementation to write unit tests compatible with JNDI, servlet, portlets. With Integration Test, this module supports loading beans according to different contexts.
8. Applying Spring to individual Java projects
I used to develop a CLI interface product – Command Line Interface – command line interface. This product has Java-based business rules engine and is very heavy on XML parsing. When the system has a problem, it's hard to test where the problem is happening.
The solution I proposed at the time was to apply Spring Framework to the test process. I use Spring Context, Spring Test and JUnit modules, then create a test package in that project. Tester will have an XML file and unit tests to prepare the data before each test.
9. Convert application into executable form with Spring boot:
Spring Boot is a new project based on the idea of using Spring itself to simplify Spring.
Spring Boot mainly uses automatic configuration to eliminate traditional Spring configuration. Whether we use Gradle or Maven, Spring Boot provides some pre-optimized starter projects to reduce the build file size.
10. Spring Social and the process of integrating media
I used this module in my startup a few years ago (my startup failed – but at least not because of technological reasons)
I use Spring Social to allow users to sign in to their application. My application allows login from Facebook, Google, LinkedIn, Twitter … .. Spring Socical uses OAuth mechanism to make login from social networking sites smooth.
I also apply Spring Mobile to my application. Spring Mobile allows you to fine tune the application to suit mobile devices. However, the fact that Spring Mobile is not the best choice for mobile devices. Instead, consider Bootstrap and AngularJS.
11. Start a project with Spring
Spring will help you start any project that suits your own needs.
Within minutes, Spring Boot will help you create a web app or standalone app, which is simple but no less powerful. You can use Grails, the results are similar!
Spring Cloud is the foundation for building micro service quickly. Micro service built by Spring Cloud has been automatically configured for resgistration and discovery.
Spring XD will be an effective assistant for distributed systems and big data.
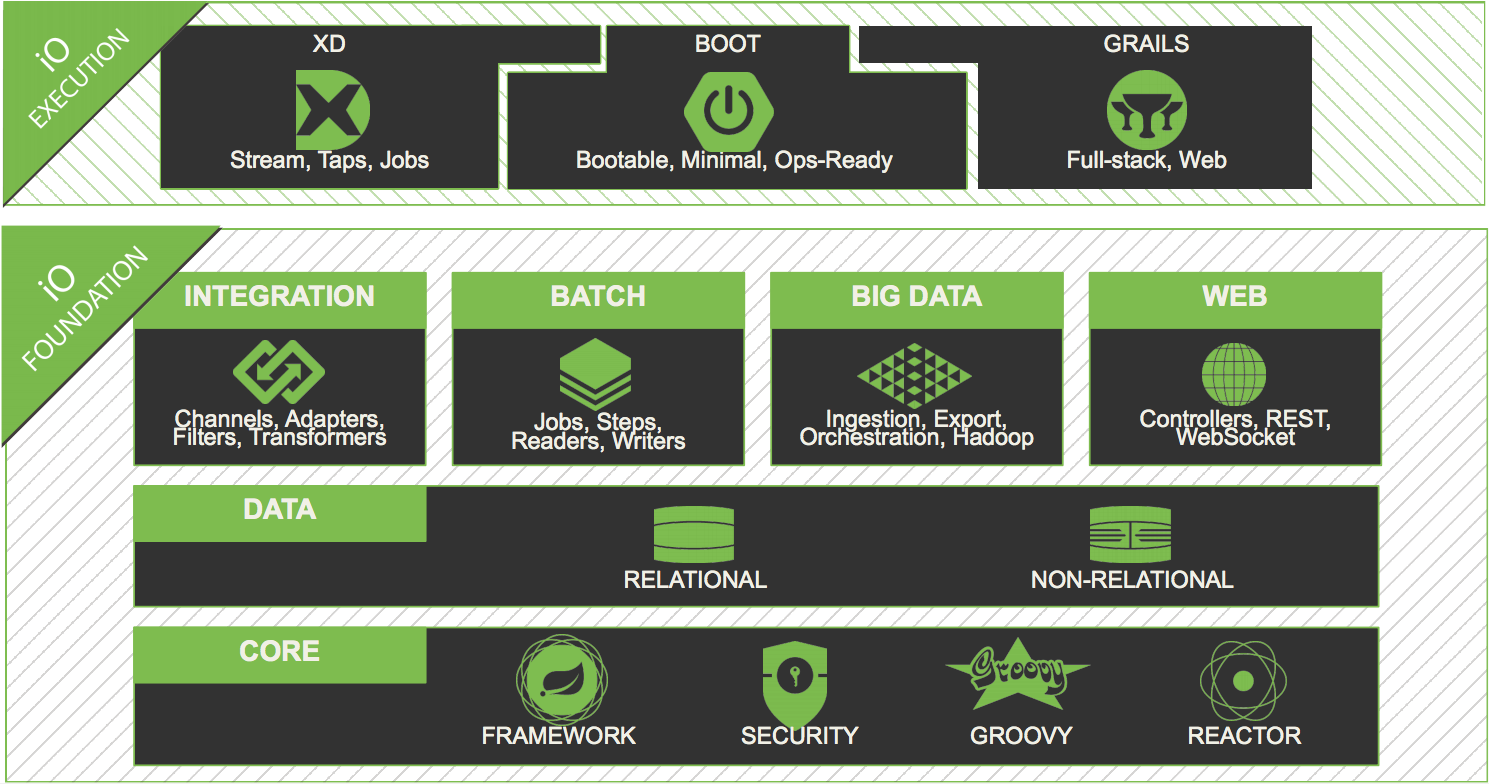
summary
I hope this article has helped you understand the basic concepts of operating mechanism as well as components of Spring Framework.
For the issues that I have mentioned, if you find other solutions, please share with me and the developer community around the world.
ITZone via Techmaster